Inside the Modal Code Playground
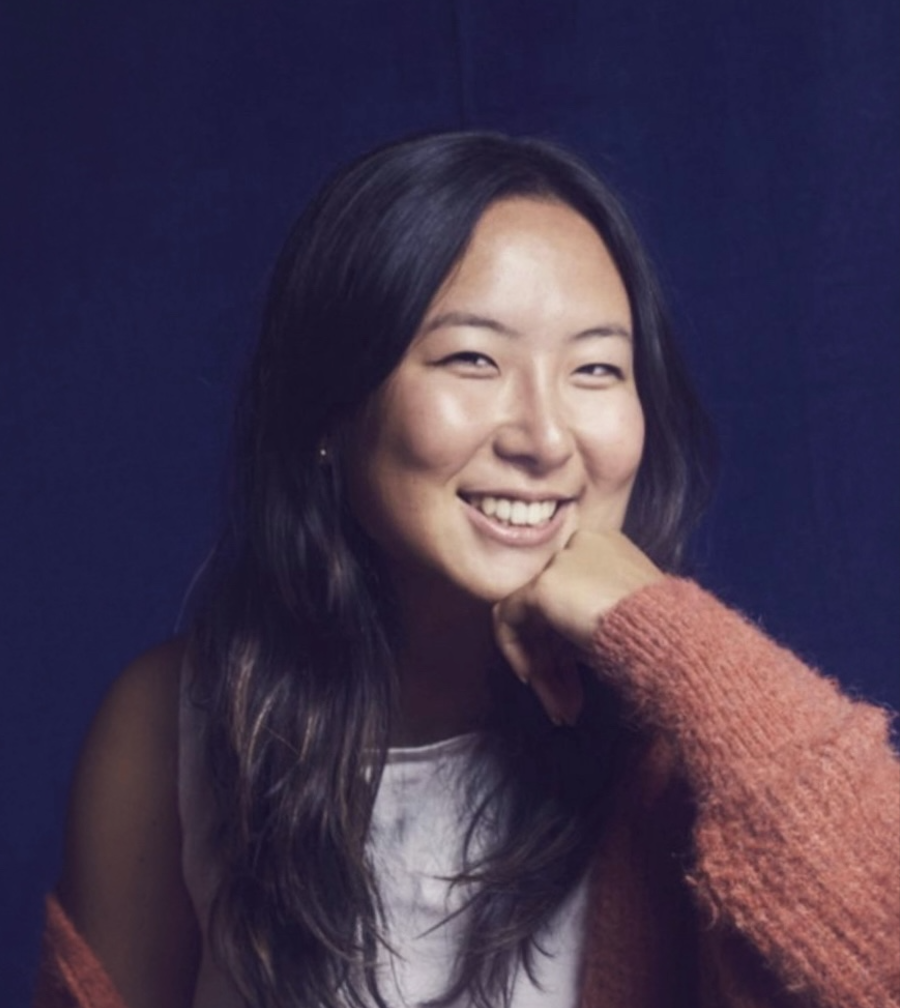
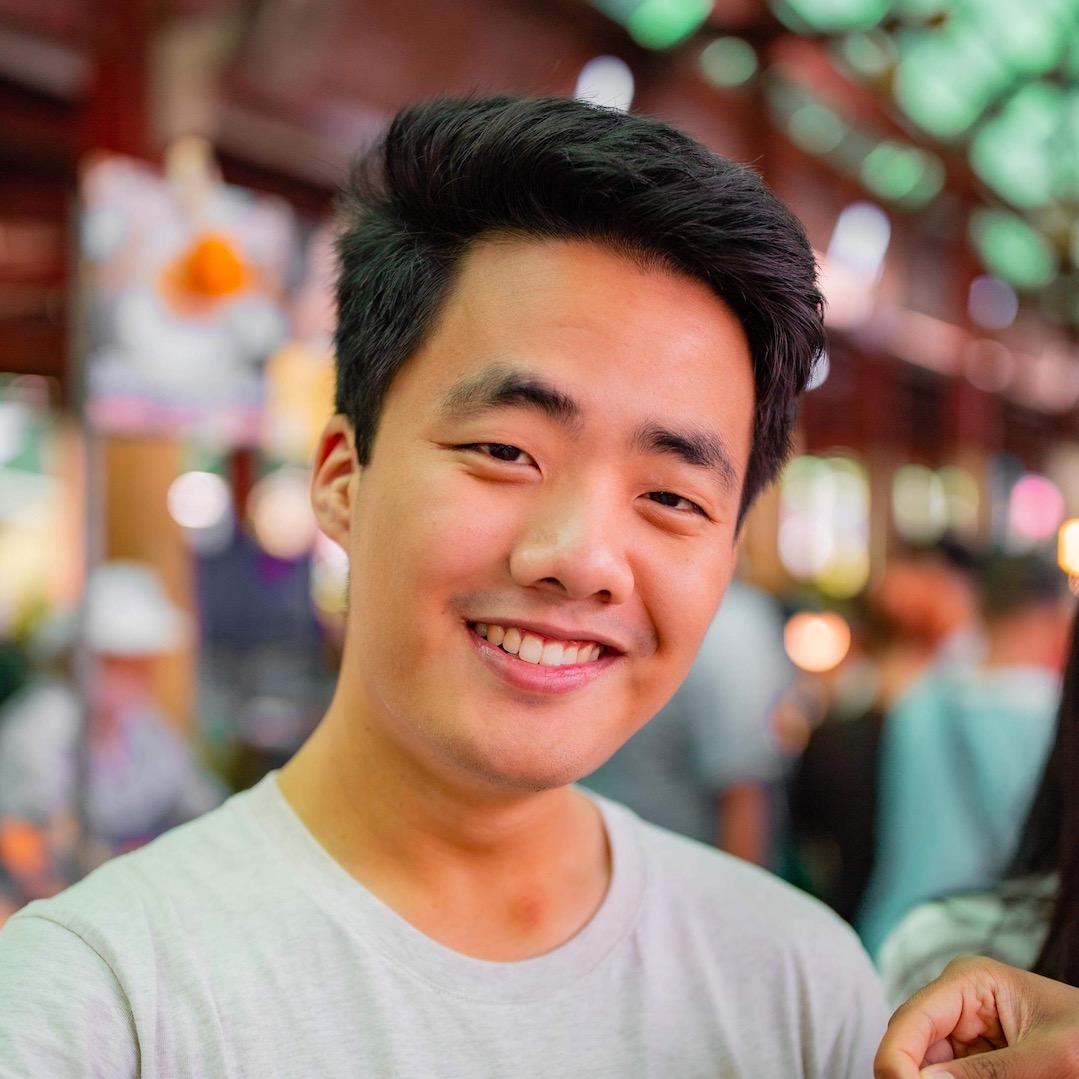
At Modal, our mission has always been to make running code in the cloud super fast and simple. With our new interactive code playground, we just made this even easier—anyone can write and run code on Modal directly from their web browser. Curious developers can try Modal before installing it, and even experienced Modal users can conveniently explore new features and experiment.
For new users, we’ve included a few interactive tutorials that introduce key Modal features and help get started with running code in the cloud. We plan on adding more to this series and expanding the playground’s functionality as we continue to extend the playground’s role in the Modal learning journey.
How does the playground work?
Let’s dive into how we quickly built an in-browser code execution environment using some of Modal’s own features.
Backend
While the backend itself is pretty straightforward, the most interesting part of our implementation is how we safely execute arbitrary user code in an isolated environment. For this, we turn to our very own Modal Sandboxes, which provide an elegant way to programatically spin up arbitrary containers, define their compute requirements, and execute code within them.
Here’s a breakdown of what happens when a user hits the “Run” button in the playground:
- Our frontend sends an HTTP request to our web server, which authenticates the request and sends a corresponding request to a Modal app deployed as a web endpoint.
- The Modal app spawns a
modal.Sandbox
with themodal
package installed and a file containing the user’s code mounted to the container. The program is executed within the sandbox withmodal run
, using the user’s ephemeral token credentials to authenticate themodal
client library and run the program in their workspace. - As the program is executed, the standard output and standard error logs are streamed back to the server. The sandbox is then torn down by default when the program is finished.
Our code execution function can be simplified to something that looks like this:
@app.function()
async def execute_user_code(code: str, user_credentials: Dict[str, str]):
with open("code.py", "w") as f:
f.write(code)
sb = await modal.Sandbox.create.aio(
"modal",
"run",
"/app/code.py",
image=modal.Image.debian_slim().pip_install("modal"),
mounts=[modal.Mount.from_local_file("code.py", "/app/code.py")],
secrets=[modal.Secret.from_dict(user_credentials)],
app=app,
)
async for line in sb.stdout:
yield line
await sb.wait.aio()
This approach allows us to safely and efficiently execute untrusted code in the user’s Modal workspace, and enable the user to access to all the compute that Modal has to offer. Implementing all of this infrastructure took just a few lines of Python!
Frontend
Luckily, to build a rich in-browser programming interface, we didn’t have to start from scratch. We leveraged a couple Javascript libraries, including CodeMirror for our code editor and Xterm.js for our integrated terminal. For now, we just stream back the sandbox output to the emulated console, though we plan on enabling some version of a CLI interface in the near future.
Limitations
While the playground is a great scratchpad and place for learning Modal basics, it’s important to note some limitations with running code in such a restricted environment. Modal is designed to make remote development feel local, so obviously when your “local environment” actually a remote Debian Linux container with just a basic Python installation and the modal
client library, and the only CLI command you can execute is modal run
, there’s a lot of cool Modal functionality that you’ll miss out on.
To unlock the full potential of Modal, including features such as iterating on and deploying web endpoints, adding local files to your custom Images, and opening a shell in running containers, we recommend downloading the Python package and setting up Modal locally:
pip install modal
python3 -m modal setup
Conclusion
We’re excited to see how this playground helps more users explore and experiment with Modal. Users can also consult our guides to dive deep into Modal features and best practices, and examples to see how to combine all of these features for their specific use case.
Get started with the Modal playground today!